What is a function – How to declare it ?
A function is a block of organized, reusable code that is used to perform a single, related action. Functions provide better modularity for your application and a high degree of code reusing. For example, in my last article, we wrote a program which was able to calculate the number of even numbers in a specific range. If you want to reuse the same code multiple times in your code, the best way is to use functions.
- The definition of a Python function begins with the keyword def followed by the function name and parentheses ( ( ) ).
- Any input parameters or arguments should be placed within these parentheses. You can also define parameters inside these parentheses.
- The code block within every function starts with a colon (:) and is indented.
- The statement return [expression] exits a function, optionally passing back an expression to the caller. A return statement with no arguments is the same as return None.
Here is a basic example :
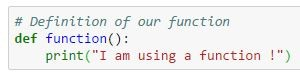
Now, if we want to use it, we have to call it :
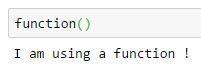
Now let’s use parameters !
As explained previously, we can add parameters in our definition and use them in the function :
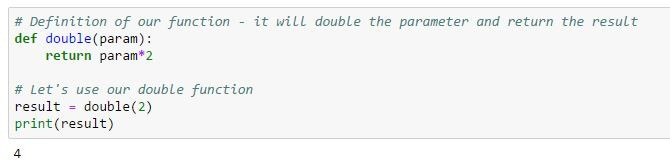
As we can see, the function returns “4” which is 2 times 2 !
Finally, an example with multiple parameters :
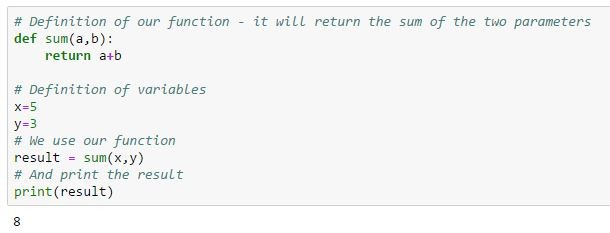
Exercise
Subject
In this exercise, we have the following people :

We will have to create a function which will calculate the Body Mass Index (BMI) and will return the BMI category based on two parameters (height in meters and weight in kilograms)
Here is the formula you will use to calculate the BMI :
BMI = weight (kg) ÷ height^2 (m)
And here is what will help you to determine in which BMI category they are :
- BMI < 20 : Underweight
- 20 < BMI < 25 : Healthy
- BMI > 25 Overweight
Solution
Multiple solutions are possible, here is one :
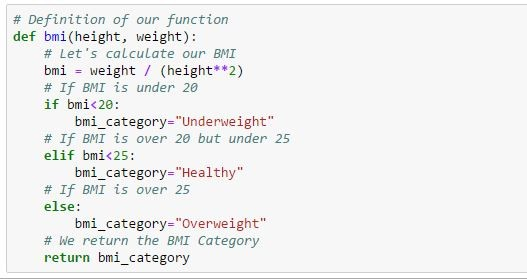
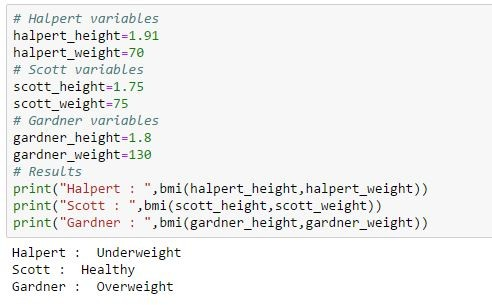
In my next article we will see together how to use Dictionnaries in Python !