Data Collections Types
There are four collection data types in the Python programming language:
- List is a collection which is ordered and changeable. Allows duplicate members.
- Tuple is a collection which is ordered and unchangeable. Allows duplicate members.
- Set is a collection which is unordered and unindexed. No duplicate members.
- Dictionary is a collection which is unordered, changeable and indexed. No duplicate members.
In this article, we will only take a look at Lists and Dictionnaries. They are the most used Data Collections Types.
Lists in Python
Lists are like arrays in other languages such as Java or PHP. It’s a type of data which allows you to store multiple values.
How to declare a list
In the following code, we will store different values in two lists and print them :
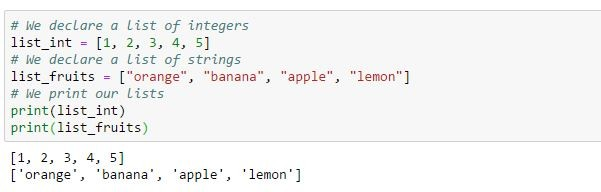
Pretty easy, isn’t it ?
Operations on lists
In this example, you can find different operations on our lists :
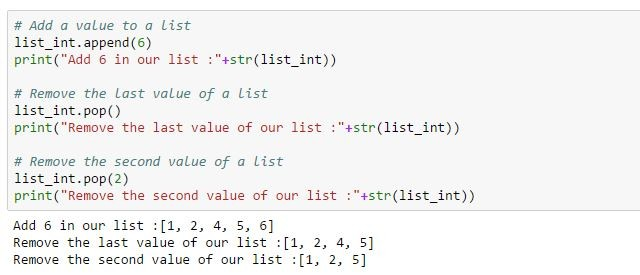
Access Data on lists
In this example, you can see how to access to a specific value in a list :

Exercise
Could you switch 2 values in a list ? For example in our list_fruits, could you change “orange” and “lemon” ?
Here is the list of all Python List Methods : Link
Here is a possible solution :
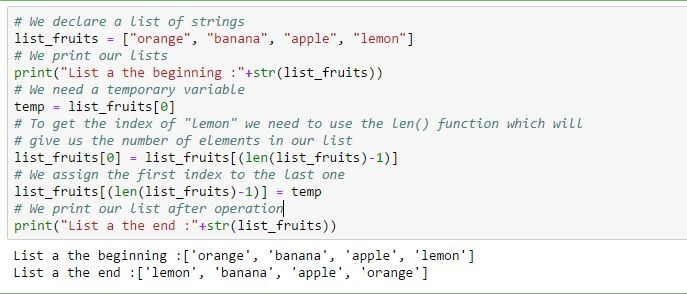
Dictionaries in Python
A Python dictionary is a mapping of unique keys to values. Dictionaries are mutable, which means they can be changed. The values that the keys point to can be any Python value. Dictionaries are unordered, so the order that the keys are added doesn’t necessarily reflect what order they may be reported back.
How to declare a dictionary
In this example, we declare a dictionary with 2 informations, the name and the age of 3 different people:

Operations on dictionaries
Here is the list of Python Dictionaries Methods : Link
You can see different operations on our dictionary in the following example :
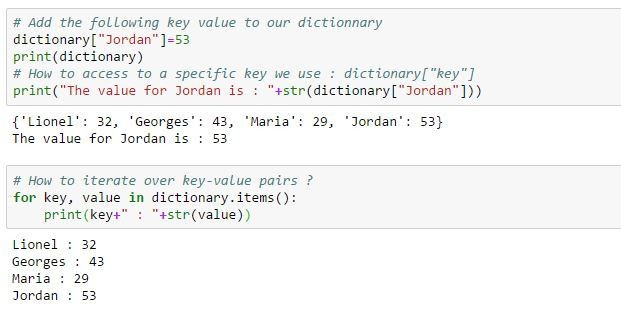
That’s it, you know how to use lists and dictionnaries in Python !
In the next article, we will see together how to use Classes and Objects !