Introduction
In mathematics and computer science, an algorithm is an unambiguous specification of how to solve a class of problems. Algorithms can perform calculation, data processing, and automated reasoning tasks. Some algorithms are better than others even if they produce equal results. Generally, the fewer steps it takes to compute, the better it is. But sometimes we care about other factors, like how many memory it uses.
Here is the list of the 32 most famous algorithms used in computer Science : Link
Let’s begin with most used Sort Algorithms !
Sorting is the most heavily studied concept in Computer Science. …
Computers sort all the time. Looking for the cheapest ticket, arranging your email by most recently sent, or sorting your contacts by last name.
There are so many algorithms to sort Data :
- adaptive sort
- quick sort
- merge sort
- even bubble sort or spaghetti sort ! (not kidding)
- etc.
We will just look at the most famous one in this article.
Heap sort
Time to execute : nlog(n) (where n is the number of element)
A heap sort algorithm is a sorting technique that is based exclusively upon a binary heap data structure. It involves finding the largest (maximum) element and sorting it at the end of an unsorted collection.
Here are the different steps used to sort the list :
- We have an unsorted array :
For example : 4, 10, 3, 5, 1
- We transform that unsorted array into a heap (or a tree) :
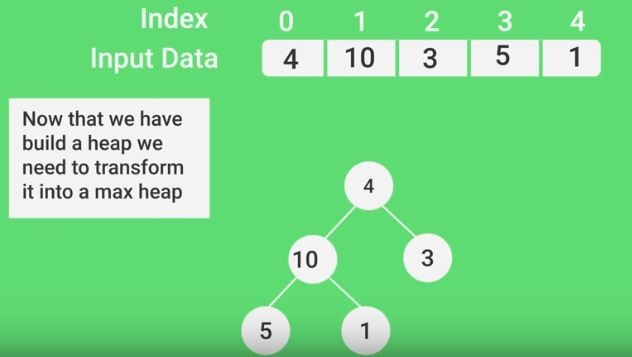
2. We transform that heap into a max heap by switching values. Parent node is always greater than or equals to child nodes.
- We start from the bottom.
- 10 is already larger than 5 and 1 so we move on.
- 4 is larger than 3 but smaller than 10, We switch 10 and four :
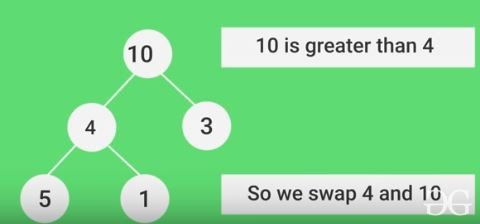
- Same thing with 4 and 5 :
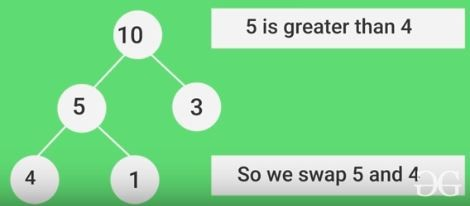
Our array (4, 10, 3, 5, 1) becomes (10,5,3,4,1)
3. We move 10 to then end of our array (we know it will be the max number of our array) and swap the smallest at the top. So we have now :
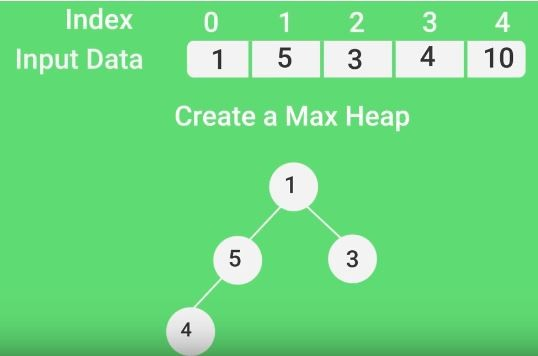
4. Then, we create a new Max Heap as explained previously until our array is sorted !
Full example :
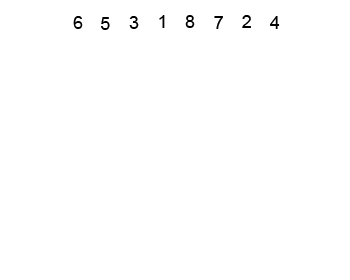
Quick Sort
This sorting algorithm the idea of divide and conquer. It finds the element called Pivot in such a way that elements in the left half are smaller than pivot and elements in the right are greater than pivot.
The three easy following steps are performed recursively :
- Bring the pivot to its appropriate position such that left of the pivot is smaller and right is greater.
- Quick sort the left part.
- Quick sort the right part.
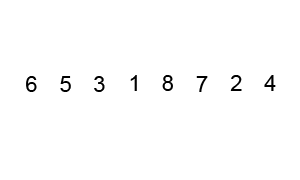
Merge Sort
As the Quick sort, the Merge sort is a divide and conquer algortithm.
It divides input array in two halves, calls itself for the two halves and then merges the two sorted halves.
Pretty easy, and very efficient as explained in the following video :
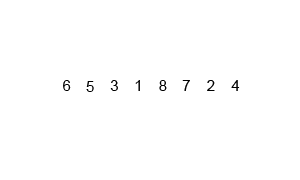
In the next article we will see together how Search Algorithms work !