Python is an object oriented programming language. Almost everything in Python is an object, with its properties and methods. A Class is like an object constructor, or a “blueprint” for creating objects.
In this tutorial we will create a basic Class called “Car” with different properties and methods.
Let’s define what our Class will do
First, let’s decide what our Class will do and what properties it will have.
We want to create a Class “Car”. We can for example define the following properties :
- Brand
- Model
- Color
This Class will have different basic methods like :
- GetBrand
- GetModel
- GetColor
Let’s declare our Class with parameters
If you know Java, you can consider the “self” in the next image as a “this”. (Or $this in PHP, or @ in Ruby 🙂 )
If you don’t know Object oriented programming don’t worry ! It’s not difficult.
Basically, in this example, we create a Class “Car”. The first function we have to create is the function called “constructor” __init__.
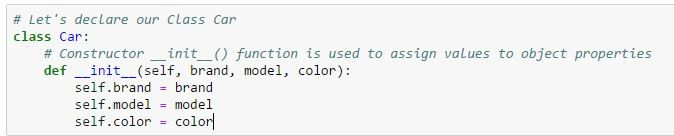
You can see 4 different parameters. The first one, “self”, is mandatory and refers to the object itself. We don’t need to go deeper on the subject, just remember that’s mandatory and it refers to our object. Then, you can see that we assign values to object properties. It’s how our object is initialize.
We can now create a “Car” object :

But as we don’t have functions yet, this object is useless.
Let’s create functions for our Class
Here is the code I have used to create the 3 functions explained in the first part of this article :
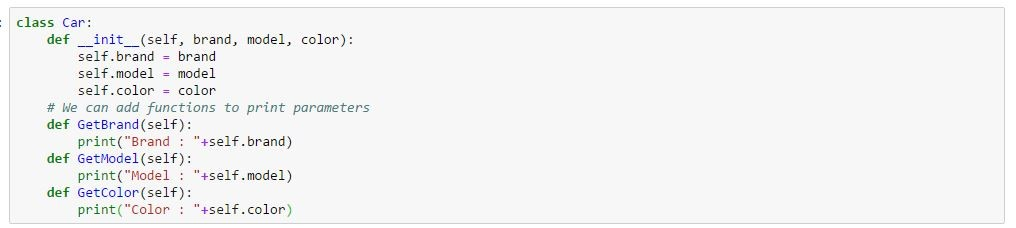
We have 3 functions which basically display the brand, the model or the color of our object “Car.”
Let’s use that Class on it functions in an example :

That’s it, we have a functional Class with parameters and functions.
Exercise
Questions
- You will use the “Car” Class to create two objects : a Black A4 Audi and a White X1 BMW.
- You will create a “Person” Class with the following attributes :
- Name
- CarOwned
3.You will use the “Person” Class to create two objects :
- Steve who owns the Audi
- John who owns the BMW
4. Without adding a new function, you will print the color of the car owned by Steve
Solution :
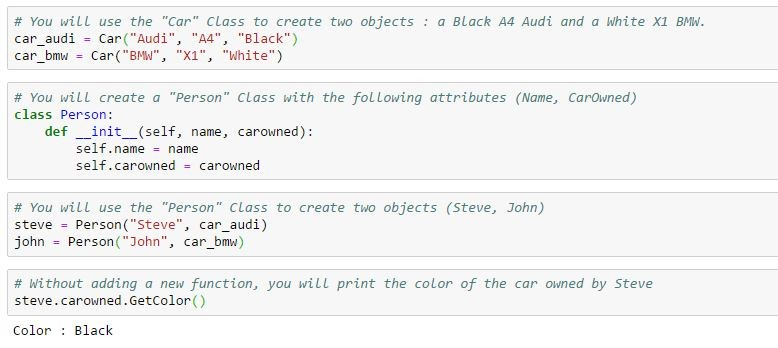
That’s it ! You know how to use Class in Python !