Requirements
In order to test the following tutorial, please setup a Python development environment as explained in my last post : link
Print and Variables
One of the most useful function in development is to be able to print values.
Here is how you can print a simple text with Python :
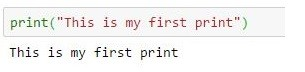
Variables are used to store values. In this example, variable “a” will refer to the value 1. Then we print it :
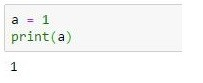
Python allows you to perform mathematical operations such as sums :
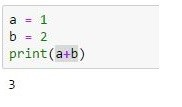
However, we have to be aware of what type of variable we are using. In our previous cased we used 2 integers. What happens if we add two strings together :
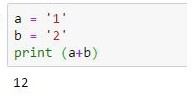
As you can see, we concatenates the 2 strings.
If we want to concatenate a string and an integer, we have to specify and force the type of our variable. In that case, we change temporary the type of variable of integer “a”, to a string :
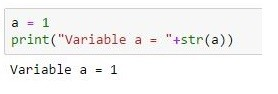
Let’s know find a way to switch between to variables their values. We will have to use a temporary variable :
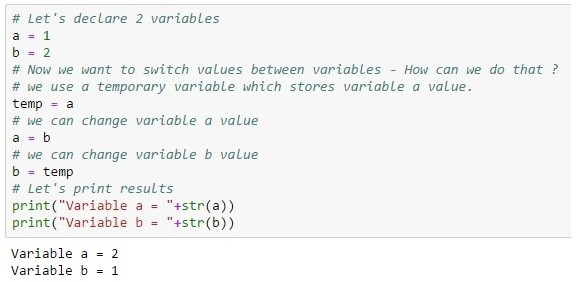
We can also create lists or range of integers with the following syntax :
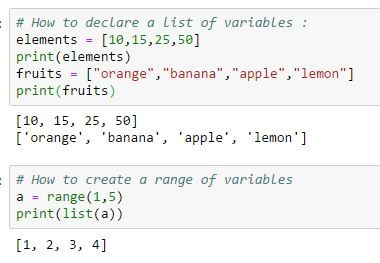
With this example, I hope you better understand variables and prints. 🙂
Now, let’s see how to use statements.
Statements
In this example, we will compare variables and use “if” and “else” statements :
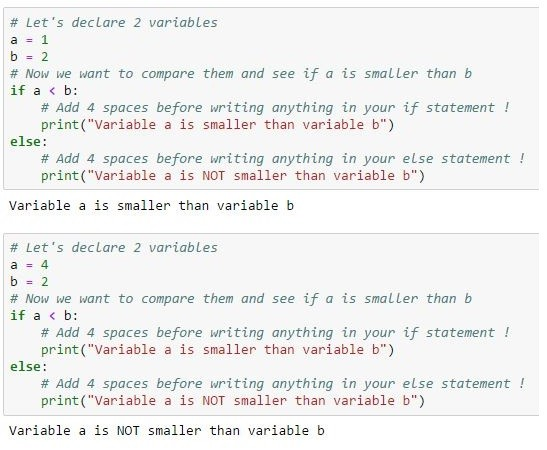
But how can we handle a third case, like “if a equals b” ?
Well, you can add a “if” in your “else” statement :
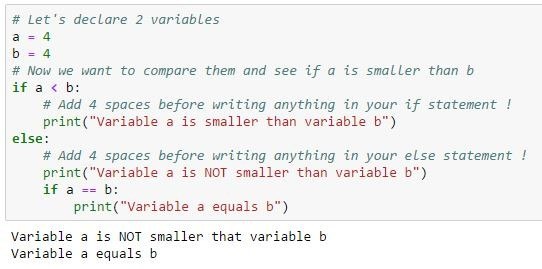
But the best way in my opinion is to use an “elif” (or “Else if” if you prefer)
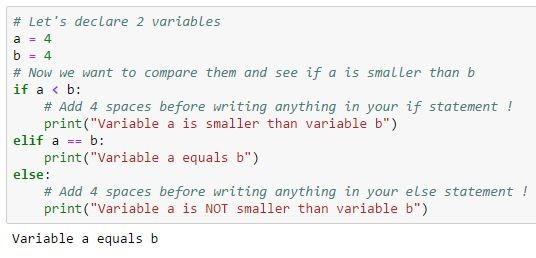
Loops
Loops allow you to perform multiple operations.
For example, in that case we have a list of values. Without a loop, we have to be able to print each value, call multiple “print” functions :
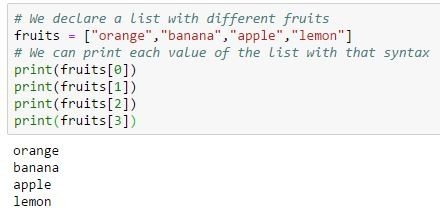
But with a “for” loop, things are handle easily :
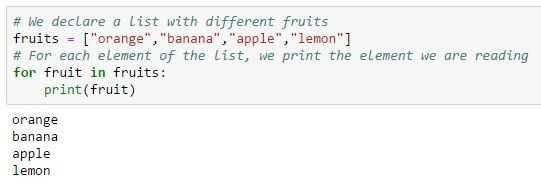
In that example, we will try from a list of integer, calculate the sum :
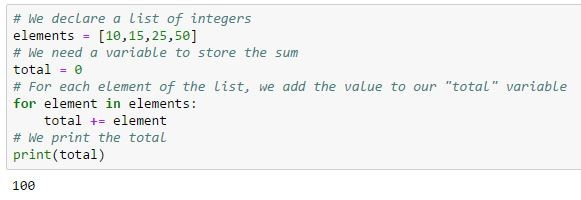
The last loop we will take a look at today is the “while” loop. It allows you to perform actions multiple times until a state is reached. In that case, we will program a countdown which will decrease an integer :
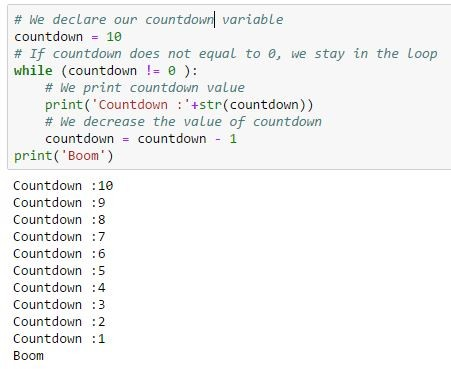
Let’s finish with a little task using what we just learnt !
Okay, now let’s use what we have learnt with a little task.
How could we count how many numbers are even in the range 1-100 ?
FYI, the way to know if a number is even or odd is the use a modulo operator :
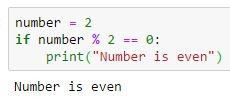
If it return 0, it means that the division of our number by 2 has a remainder equals to 0. So it can be divided by 0 !
Solution :
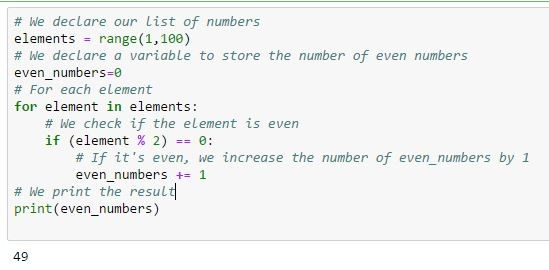
That’s all for today !
In my next Linkedin article, we will see how to use Functions with Python !